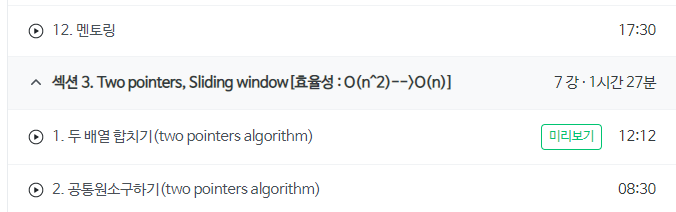
모각코 1회차etc./모각코2022. 7. 6. 19:00
Table of Contents
- 알고리즘 문제 풀이
1. 배열 - 격자판 최대합
package inflearn.array;
import java.util.Arrays;
import java.util.Scanner;
public class P02_09 {
public static int solution(int n, int[][] arr) {
int answer = Integer.MIN_VALUE;
// 먼저 각 행, 열의 합에서 최대 값을 찾는다.
int sumRow, sumCol;
for (int i = 0; i < n; i++) {
// 각 행, 열의 합계 초기화
sumRow = 0;
sumCol = 0;
// 한번에 행과 열의 합계를 같이 구한다.
// 반복하며 어떤 변수가 변하느냐에 따라 행, 열 여부가 결정된다.
for (int j = 0; j < n; j++) {
sumRow += arr[i][j];
sumCol += arr[j][i];
}
// 세 값 중에 가장 큰 값을 answer로 선택한다.
answer = Math.max(sumCol, Math.max(answer, sumRow));
}
// 마지막으로 대각선의 합을 구한다.
int sumA = 0, sumB = 0;
for (int i = 0; i < n; i++) {
sumA += arr[i][i];
sumB += arr[i][n - i - 1];
}
// 세 값 중에 가장 큰 값을 answer로 선택한다.
answer = Math.max(sumA, Math.max(answer, sumB));
return answer;
}
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
// 2차원 배열 입력받기
int[][] arr = new int[n][n];
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
arr[i][j] = sc.nextInt();
}
}
System.out.println(solution(n, arr));
}
}
2. 배열 - 봉우리 개수 세기
package inflearn.array;
import java.util.Scanner;
public class P02_10 {
public static int solution(int n, int[][] arr) {
int answer = 0; // 봉우리 개수
// 상하좌우 탐색할 index를 미리 정해놓는다. -> for-loop으로 간단하게 처리가능
int[] dx = {0, 0, 1, -1};
int[] dy = {-1, 1, 0, 0};
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
/*
// 전체를 순회하며 상하좌우보다 값이 큰 원소를 카운트한다.
// 각 인덱스가 가장자리에 위치하면 항상 봉우리가 될 가능성이 있으므로 이 경우 바로 다음 조건을 검사한다.
if (i-1 >= 0 && arr[i][j] <= arr[i-1][j]) continue;
if (i+1 < n && arr[i][j] <= arr[i+1][j]) continue;
if (j-1 >= 0 && arr[i][j] <= arr[i][j-1]) continue;
if (j+1 < n && arr[i][j] <= arr[i][j+1]) continue;
// 여기에 도달하면 arr[i][j]는 봉우리다.
answer++;
*/
boolean flag = true;
for (int k = 0; k < 4; k++) {
int nx = i + dx[k];
int ny = j + dy[k];
if(nx >= 0 && nx < n && ny >= 0 && ny < n && arr[nx][ny] >= arr[i][j]) {
flag = false;
break; // 하나라도 크거나 같다면 이미 arr[i][j]는 봉우리가 아님
}
}
if (flag) answer++;
}
}
return answer;
}
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
// 2차원 배열 입력받기
int[][] arr = new int[n][n];
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
arr[i][j] = sc.nextInt();
}
}
System.out.println(solution(n, arr));
}
}
3. 배열 - 임시 반장 정하기
package inflearn.array;
import java.util.Scanner;
public class P02_11 {
public static int solution(int n, int[][] arr) {
int answer = 0;
int max = Integer.MIN_VALUE;
// 학생 수 찾기
for (int i = 1; i <= n; i++) {
int count = 0;
for (int j = 1; j <= n; j++) {
if (i == j) continue; // 자기 자신과는 비교하지 않음
for (int k = 1; k < 5; k++) {
if (arr[i][k] == arr[j][k]) {
count++;
break; // 전체 학년 (k)를 통틀어 한 번이라도 같은 반이 되었다면 그 한번만 카운트한다.
}
}
}
if (count > max) {
max = count;
answer = i;
}
}
return answer;
}
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
// 2차원 배열 입력받기
int[][] arr = new int[n+1][6];
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= 5; j++) {
arr[i][j] = sc.nextInt();
}
}
System.out.println(solution(n, arr));
}
}
@gmelon :: gmelon's greenhouse
백엔드 개발을 공부하고 있습니다.